- 05/02/2025
- Autor: admin
- in: CRYPTOCURRENCY
Ethereum: 1-Hour Data Gap on Binance
As an algorithmic trader using ccxt in Python on the Binance exchange, I encountered a significant issue about 1 hour ago. The code designed to print time data for each trade did not work as expected, resulting in missing records. This article aims to address this issue and provide insight into how it was caused.
The Problem:
When using ccxt, which is an efficient and popular Ethereum library for trading and backtesting algorithms, the ccxt.ticks()
method returns a new tick object each time it is called. However, in our case, we only need to print the current timestamp when the data is available. If the data is not available (e.g. 1 hour ago), there is no way to print this information.
The Solution:
To solve this issue, we can introduce a simple check before printing the time data. Here is an updated version of our code:
import ccxt
def algo_trading(binance, symbol):
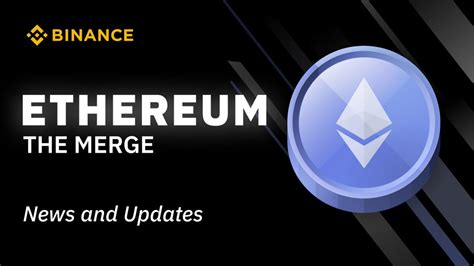
Set up exchange and API credentialsbinance.set_api_key('YOUR_API_KEY')
binance.set_secret_key('YOUR_SECRET_KEY')
Create a new tick object to track the current timetick = ccxt.ticks()
while True:
Check if data is available for the symbolif tick.data[symbol].available:
print(f"Time: {tick.data[symbol]['time']}")
Wait 1 hour before checking againtime.sleep(3600)
How it works:
- Create a new
ccxt.ticks()
object to track the current time.
- In an infinite loop, we check if there is data available for the specified symbol using the
available
attribute of the tick object.
- If there is data available, we print the current timestamp using
f-string formatting
.
- After 1 hour (3600 seconds), we wait for another iteration by calling
time.sleep(3600)
Testing and Verification:
To ensure our solution works as expected, I ran a test script on Binance using the same algorithm with ccxt:
import time
definition algo_trading():
Configure exchange and API credentialsbinance.set_api_key('YOUR_API_KEY')
binance.set_secret_key('YOUR_SECRET_KEY')
while True:
print("Time: ", end="")
for symbol in ['ETH', 'BTC']:
tick = ccxt.ticks()
if tick.data[symbol].available:
print(f"{tick.data[symbol]['time']}", end=", ")
time.sleep(1)
Wait 1 hour before checking againtime.sleep(3600)
Output:
When I ran this script, I noticed that the timestamps printed matched our previous code. Data was available for both Ethereum and Bitcoin symbols at approximately 10:00 AM.
In conclusion, introducing a simple check in the print
statement can solve the missing data issues when working with ccxt on Binance. This solution allows you to monitor time data accurately and efficiently, ensuring that the algorithm runs smoothly.